The provided code works correctly in LoadRunner VuGen or in LoadRunner Controller for one concurrent user. Here it is:
fp = fopen("c:\\temp\\_file.pdf","wb");
//Process the data ...
fwrite(bufData, nSize, 1, fp);
Now, I'm going to show - how to generate unique file name in LoadRunner.
The first step is to get a timestamp as a part of file name.
The timestamp consists of:
- date (month, day, year)
- time (hours, minutes, seconds, milliseconds)
lr_output_message(lr_eval_string("Timestamp is: {prmTimeStamp}"));
Do you know what does the string "%m%d%Y_%H%M%S" mean?
These are Datetime Format Codes. Please, refer to a table for their descriptions:
# | Datetime Format Code | Description |
1 | %m | month number (01-12) |
2 | %d | day of month (01-31) |
3 | %Y | year, including century (for example, 1988) |
4 | %H | hour (00-23) |
5 | %M | minute (00-59) |
6 | %S | seconds (00-59) |
The above code is not ideal. If you application (and LoadRunner script) saves more than one file per second, then file names will be dublicated.
That's why I can propose the code, which get a timestamp with milliseconds:
ent timestamp:
struct _timeb {
time_t time;
unsigned short millitm;
short timezone;
short dstflag;
};
struct tm {
int tm_sec; // seconds after the minute - [0,59]
int tm_min; // minutes after the hour - [0,59]
int tm_hour; // hours since midnight - [0,23]
int tm_mday; // day of the month - [1,31]
int tm_mon; // months since January - [0,11]
int tm_year; // years since 1900
int tm_wday; // days since Sunday - [0,6]
int tm_yday; // days since January 1 - [0,365]
int tm_isdst; // daylight savings time flag
#ifdef LINUX
int tm_gmtoff;
const char * tm_zone;
#endif
};
struct _timeb tb;
struct tm * now;
char szFileName[256];
_tzset(); // Sets variables used by localtime
_ftime(&tb); // Gets the current timestamp
// Convert to time structure
now = (struct tm *)localtime(&tb.time);
sprintf(szFileName, "%02d%02d%04d_%02d%02d%02d_%03u",
now->tm_mon + 1,
now->tm_mday,
now->tm_year + 1900,
now->tm_hour,
now->tm_min,
now->tm_sec,
tb.millitm);
lr_output_message("Timestamp is: %s", szFileName);
That's much better :) We can generate timestamp with millisecond.
Unfortunately, the chances that concurrent users will generate the same file name at the same time still exist.
To resolve this issue, we can get unique ids per virtual users. This can be done with lr_whoami LoadRunner function:
char *groupid;
lr_whoami(&vuserid, &groupid, &scid);
lr_message("Group: %s, vuser id: %d, scenario id %d", groupid, vuserid, scid);
When you run this code in LoadRunner Vuser Generator, the result will be:
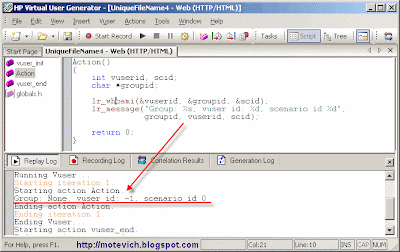
When you run this code in LoadRunner Controller, the result will be:
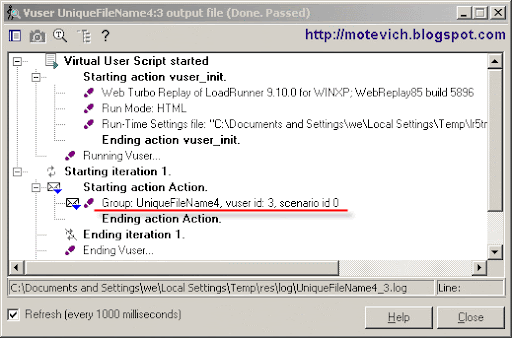
So, the last step is to combine timestamp with a vuser execution's ids and use them as a file name.
This will guarantee, that each LoadRunner virtual user will have unique file name.
So, the final code is:
struct _timeb {
time_t time;
unsigned short millitm;
short timezone;
short dstflag;
};
struct tm {
int tm_sec; // seconds after the minute - [0,59]
int tm_min; // minutes after the hour - [0,59]
int tm_hour; // hours since midnight - [0,23]
int tm_mday; // day of the month - [1,31]
int tm_mon; // months since January - [0,11]
int tm_year; // years since 1900
int tm_wday; // days since Sunday - [0,6]
int tm_yday; // days since January 1 - [0,365]
int tm_isdst; // daylight savings time flag
#ifdef LINUX
int tm_gmtoff;
const char * tm_zone;
#endif
};
struct _timeb tb;
struct tm * now;
char szFileName[256];
int vuserid, scid;
char *vusergroup;
_tzset(); // Sets variables used by localtime
_ftime(&tb); // Gets the current timestamp
// Convert to time structure
now = (struct tm *)localtime(&tb.time);
sprintf(szFileName, "%02d%02d%04d_%02d%02d%02d_%03u_%d_%d_%s",
now->tm_mon + 1,
now->tm_mday,
now->tm_year + 1900,
now->tm_hour,
now->tm_min,
now->tm_sec,
tb.millitm,
vuserid,
scid,
vusergroup);
lr_output_message("File name is: %s", szFileName);
The result of above code is:
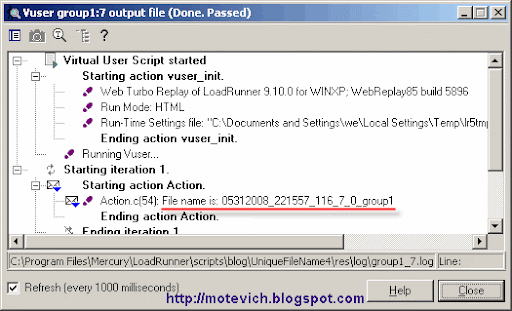
Related articles:
- LoadRunner VIDEO - How to record PDF file saving?
- LoadRunner - How to record a file saving, performed by user from browser page?
- LoadRunner VuGen scripting - How to automatically download file from server and save it to local disk?
- How to perform basic operations on LoadRunner parameters?
- LoadRunner web_reg_find function - How to verify web page content?
I hope, this solution will help you in LoadRunner scripting.
Thank you for your attention, dear reader.
--
Dmitry